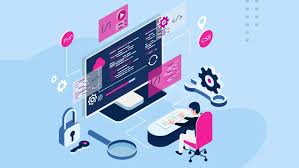
Software architecture is the fundamental organization of a software system, encompassing its components, their relationships to each other and to the environment, and the principles guiding its design and evolution. It’s the blueprint that guides the development process, ensuring the system meets its functional and quality requirements. Understanding software architecture is crucial for building robust, scalable, and maintainable software systems.
Defining Software Architecture
Software architecture is more than just code; it’s the high-level structure of a system. It defines the major components, their responsibilities, and how they interact. It also addresses key quality attributes like performance, security, and scalability.
Key Aspects of Software Architecture
- Components: These are the building blocks of the system, such as modules, services, or libraries. Each component has a specific responsibility and exposes interfaces for interaction.
- Relationships: These define how components interact with each other. Relationships can be dependencies, communication channels, or data flows.
- Constraints: These are the rules and guidelines that govern the architecture. Constraints can be technical limitations, business requirements, or design principles.
- Quality Attributes: These are the non-functional requirements of the system, such as performance, security, scalability, maintainability, and usability. The architecture must be designed to meet these attributes.
- Principles: These are the fundamental ideas that guide the design of the architecture. Principles can include separation of concerns, single responsibility, and loose coupling.
The “4+1” View Model of Architecture
A common way to describe software architecture is using the “4+1” view model, which provides multiple perspectives on the system:
- Logical View: This view focuses on the functional requirements of the system. It describes the key classes, interfaces, and subsystems. UML class diagrams (which we’ll cover in Module 2) are often used to represent this view.
- Development View: This view focuses on the software modules and their organization in the development environment. It describes how the code is structured and how it is managed. Component diagrams (which we’ll cover in Module 3) are useful here.
- Process View: This view focuses on the runtime behavior of the system. It describes the processes, threads, and communication channels. Sequence diagrams and activity diagrams (covered in Module 2) can illustrate this view.
- Physical View: This view focuses on the hardware and network infrastructure on which the system runs. It describes the nodes, networks, and deployment artifacts. Deployment diagrams (covered in Module 3) are used to represent this view.
- Use Case View (+1): This view ties all the other views together. It uses use cases (covered in Module 2) to illustrate how the system is used by its actors and to validate the architecture.
Why is Architecture Important?
A well-defined architecture provides numerous benefits:
- Reduces Complexity: By breaking down a large system into smaller, manageable components, architecture helps to reduce complexity and make the system easier to understand.
- Enables Communication: Architecture provides a common language and understanding for all stakeholders, including developers, testers, and business analysts.
- Facilitates Reuse: A well-designed architecture promotes reuse of components and patterns, reducing development time and cost.
- Improves Quality: By addressing quality attributes early in the development process, architecture helps to ensure that the system meets its non-functional requirements.
- Manages Risk: Architecture helps to identify and mitigate risks early in the development process.
- Enables Evolution: A flexible architecture allows the system to evolve and adapt to changing requirements.
Examples of Software Architecture in Practice
Let’s explore some examples to illustrate the concept of software architecture:
Example 1: E-Commerce Platform
Consider the “E-Commerce Platform” project we’ll be using as a case study throughout this course. A simplified architectural view might include the following components:
- User Interface: Handles user interactions (e.g., web pages, mobile apps).
- Product Catalog: Manages product information (e.g., name, description, price).
- Shopping Cart: Stores items selected by the user.
- Order Management: Processes orders and manages shipments.
- Payment Gateway: Handles payment processing.
- Database: Stores persistent data (e.g., products, users, orders).
The relationships between these components define how they interact. For example, the User Interface interacts with the Product Catalog to display product information, and the Order Management component interacts with the Payment Gateway to process payments.
Example 2: Ride-Sharing Application
A ride-sharing application like Uber or Lyft has a complex architecture that needs to handle many concurrent users, real-time location tracking, and payment processing. Key components might include:
- Mobile Apps (Driver & Rider): User interface for drivers and riders.
- Matching Service: Connects riders with available drivers based on location and other criteria.
- Location Service: Tracks the real-time location of drivers and riders.
- Payment Service: Handles payment processing and fare calculations.
- Notification Service: Sends notifications to drivers and riders (e.g., ride requests, arrival updates).
- Database: Stores user data, ride history, and other information.
The architecture must be highly scalable and reliable to handle peak demand and ensure a smooth user experience.
Example 3: Social Media Platform
A social media platform like Facebook or Twitter needs to handle massive amounts of data, real-time updates, and complex social interactions. Key components might include:
- Web and Mobile Frontends: User interfaces for accessing the platform.
- User Management: Handles user accounts, profiles, and authentication.
- Content Management: Stores and manages user-generated content (e.g., posts, images, videos).
- News Feed: Aggregates and displays content based on user preferences and social connections.
- Search Service: Allows users to search for content and other users.
- Recommendation Engine: Suggests content and users based on user activity.
- Database: Stores user data, content, and social connections.
The architecture must be designed for high scalability, availability, and performance to handle the large volume of data and traffic.
Hypothetical Scenario: Smart Home System
Imagine a smart home system that controls lighting, temperature, security, and appliances. The architecture might include:
- Central Hub: A device that connects all the smart home devices.
- Device Controllers: Software components that control individual devices (e.g., lights, thermostats, locks).
- Mobile App: A user interface for controlling the system remotely.
- Cloud Service: A service that provides remote access and data storage.
- Security System: A component that monitors the home for intrusions and alerts the user.
The architecture must be secure and reliable to protect the home and its occupants.
Common Architectural Styles
While we’ll delve deeper into architectural styles in Module 4, it’s helpful to briefly introduce some common styles now:
- Monolithic Architecture: A single, self-contained application. This is a simple approach but can become difficult to manage as the application grows.
- Layered Architecture: Organizes the system into layers, each with a specific responsibility. This promotes separation of concerns and makes the system easier to maintain.
- Microservices Architecture: Decomposes the system into small, independent services that communicate with each other. This allows for independent deployment and scaling of individual services.
Practice Activities
- E-Commerce Platform Components: Expand on the E-Commerce Platform example. Identify at least three more components that would be necessary for a real-world e-commerce system (e.g., a recommendation engine, a customer support module, a marketing automation system). Describe the responsibilities of each component and how it would interact with existing components.
- Ride-Sharing Quality Attributes: Consider the Ride-Sharing Application example. Identify three key quality attributes that are critical for this type of application (e.g., scalability, reliability, security). Explain how the architecture would need to be designed to meet these attributes.
- Smart Home Scenario: For the Smart Home System scenario, consider the security aspect. What architectural considerations would be important to ensure the security of the system and protect user data? Think about authentication, authorization, and data encryption.
- Architectural Style Identification: Imagine you are building a simple blog application. Which architectural style (Monolithic, Layered, or Microservices) would be most appropriate for this project, given its relatively small size and scope? Explain your reasoning.
Summary and Next Steps
In this lesson, we defined software architecture and explored its key aspects, including components, relationships, constraints, quality attributes, and principles. We also discussed the importance of architecture and examined several real-world examples. Finally, we briefly introduced common architectural styles.
In the next lesson, we’ll explore Why are Software Architecture Diagrams Important? We’ll discuss how diagrams help to visualize and communicate architectural decisions, and how they facilitate collaboration among stakeholders.